Router
How works the routing system in Graphul?​
Graphul for its router system uses the trait Method that implements a function for every HTTP Method.
We can access these methods using an instance of Graphul
or an instance of a Group
.
Besides every handler have access to the Context.
List of HTTP Methods implemented​
As we said, Graphul tries to give an implementation of every HTTP Method, here is the list:
get
post
put
patch
delete
options
trace
head
All of these are available.
If you try to access these methods directly from a Graphul
instance or a Group
instance maybe you don't see the autocompletion or maybe if you try to run it you could get an error like this:
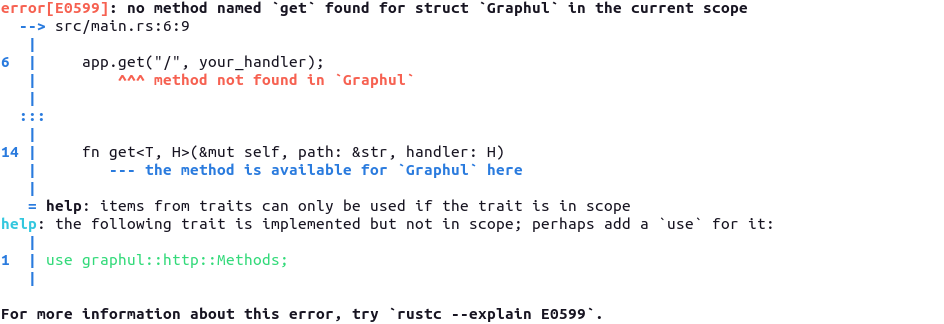
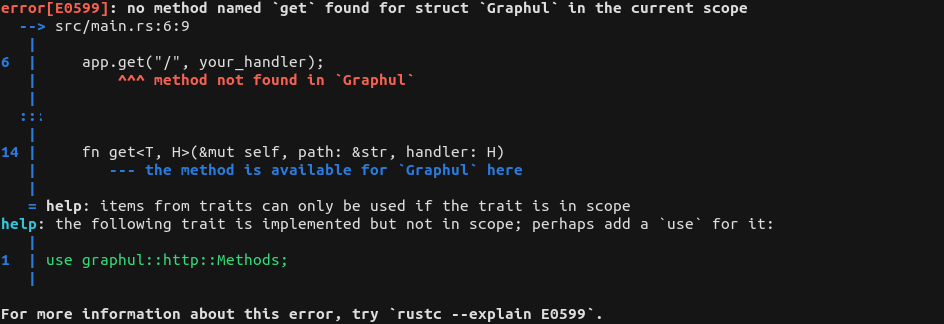
This error as said in the image is because you need to import the trait Methods
.
So you can add this line to your file and try again:
use graphul::http::Methods;
Example​
- Without Context
- Without State
- Using State
use graphul::{http::Methods, Graphul};
#[tokio::main]
async fn main() {
let mut app = Graphul::new();
app.get("/", || async { "hello world!" });
app.get("/hello", your_handler);
app.post("/hello", your_handler);
app.put("/hello", your_handler);
app.patch("/hello", your_handler);
app.delete("/hello", your_handler);
app.run("127.0.0.1:8000").await;
}
async fn your_handler() {
// ... your code
}
use graphul::{http::Methods, Graphul, Context};
#[tokio::main]
async fn main() {
let mut app = Graphul::new();
app.get("/", || async { "hello world!" });
app.get("/hello", your_handler);
app.post("/hello", your_handler);
app.put("/hello", your_handler);
app.patch("/hello", your_handler);
app.delete("/hello", your_handler);
app.run("127.0.0.1:8000").await;
}
async fn your_handler(_ctx: Context) {
// ... your code
}
use graphul::{http::Methods, Graphul, Context};
#[tokio::main]
async fn main() {
let mut app = Graphul::share_state(AppState { });
app.get("/", || async { "hello world!" });
app.get("/hello", your_handler);
app.post("/hello", your_handler);
app.put("/hello", your_handler);
app.patch("/hello", your_handler);
app.delete("/hello", your_handler);
app.run("127.0.0.1:8000").await;
}
#[derive(Clone)]
struct AppState {}
async fn your_handler(_ctx: Context<AppState>) {
// ... your code
}
Getting Path Params​
The path params are accessible with a definition of the path, for example:
- Using Path Extractor
- Using params
- With wrapper
- Without wrapper
app.get("/:name", |name: Path<String>| async move {
format!("Hi {}!", name.0)
});
app.get("/:name", |Path(name): Path<String>| async move {
format!("Hi {name}!")
});
Graphul maintains the extractors of Axum
app.get("/:name", |c: Context| async move {
let name = c.params("name");
format!("Hi {name}")
});
We declare the parameter using the :
to define a parameter and then you can access the parameter of type Context to get the query parameters inside of the handler.
Getting Query Params​
The Query Params are too similar to the Path Params.
In this case, we just call the function query
to access the Query Params.
- Using Query Extractor
- Using query
- With wrapper
- Without wrapper
app.get("/", |name: Query<String>| async move {
format!("Hi {}!", name.0)
});
app.get("/", |Query(name): Query<String>| async move {
format!("Hi {name}!")
});
Graphul maintains the extractors of Axum
app.get("/", |c: Context| async move {
let name = c.query("name");
format!("Hi {name}!")
});